- Published on
Linked List
- Authors
- Name
- Yue Zhang
TIP
- 用容器容易,用有限几个变量不容易
- 用了DummyNode就一定要返回DUMMY->NEXT
206 reverse linked list
Given the head of a singly linked list, reverse the list, and return the reversed list. 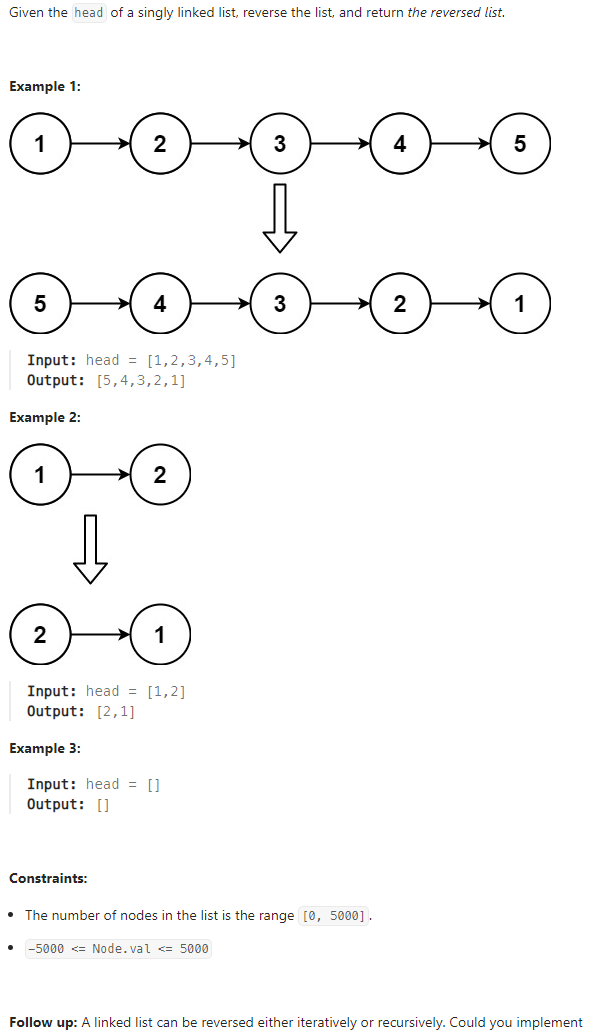
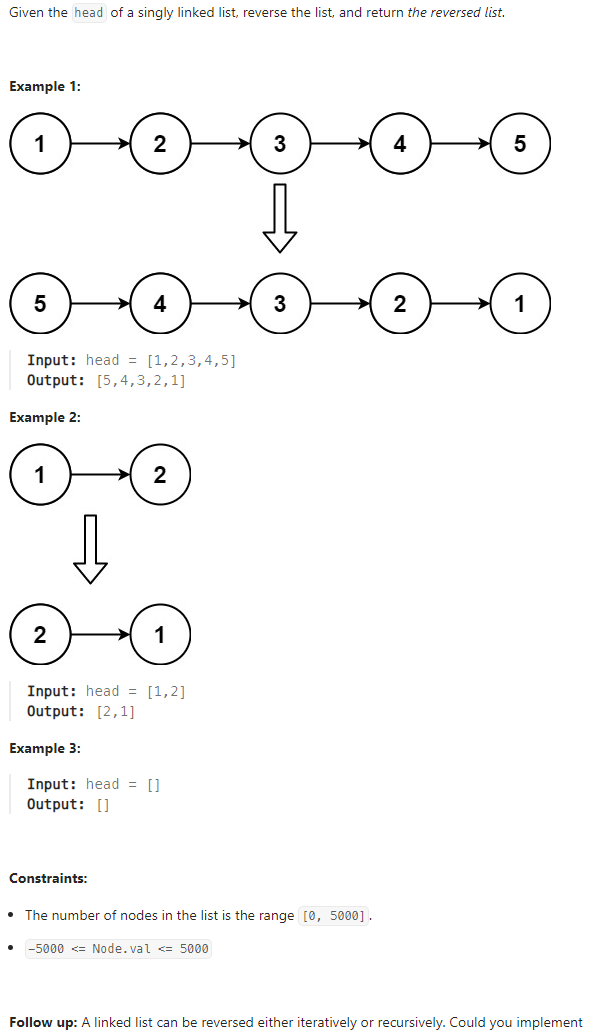
iteration
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* reverseList(ListNode* head) {
if(head == nullptr || head->next == nullptr)
return head;
ListNode* pre = nullptr;
ListNode* next = nullptr;
while(head != nullptr && head->next!=nullptr)
{
// 记录head->next
next = head->next;
// head指向prev
head->next = pre;
// prev 向后移动到head
pre = head;
// head指向next
head = next;
}
head->next = pre;
return head;
}
};