- Published on
GAMES104 02 Layered Architecture of Game Engine
- Authors
- Name
- Yue Zhang
- A Glance of Game Engine Layers
- Explore Game Engine Layers
Modern game engines are highly complex systems containing massive amounts of code. Fortunately, game engines typically organize this code through a layered architecture. Understanding the layered structure of a game engine helps form a comprehensive understanding of the entire system.
A Glance of Game Engine Layers
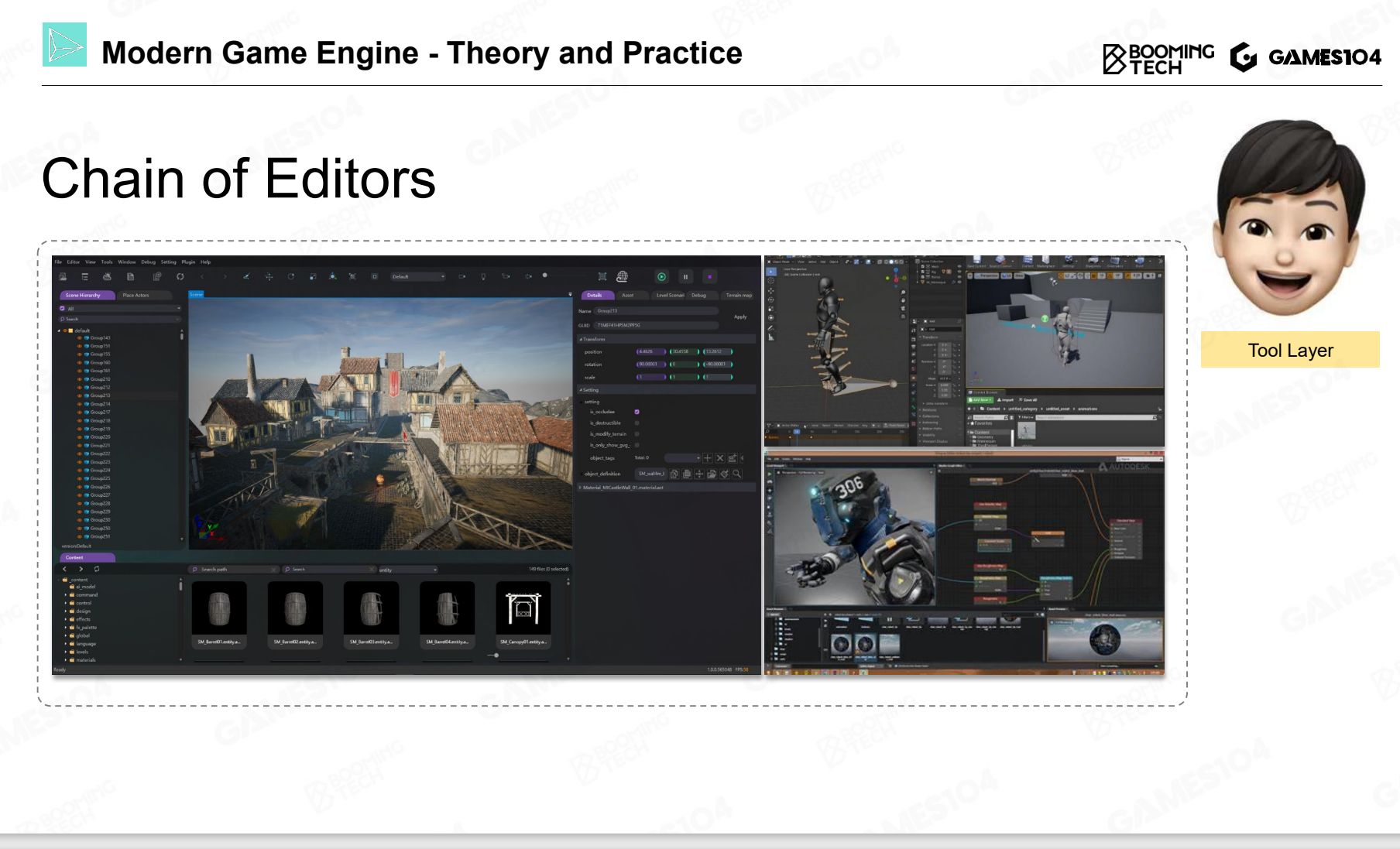
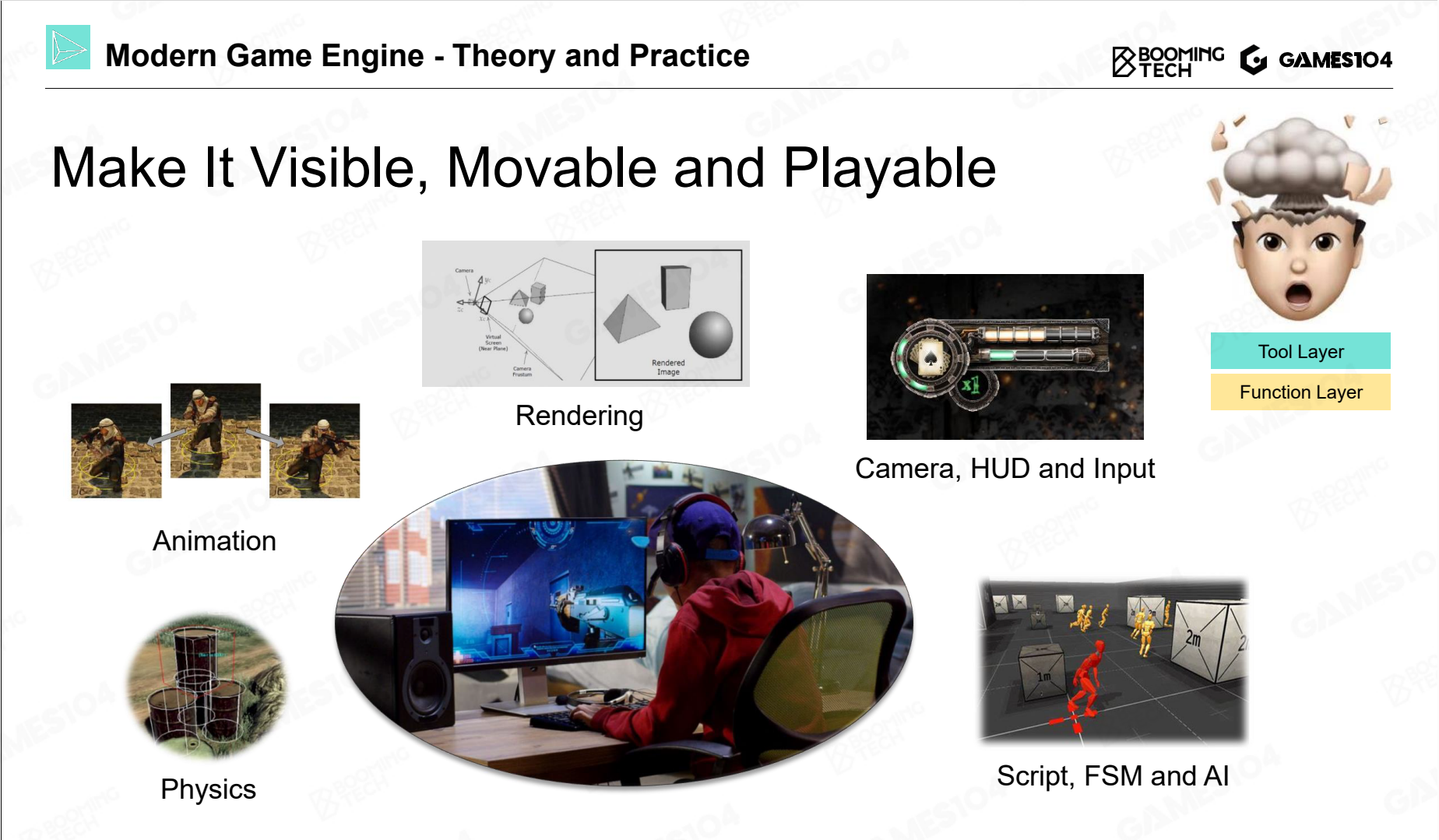
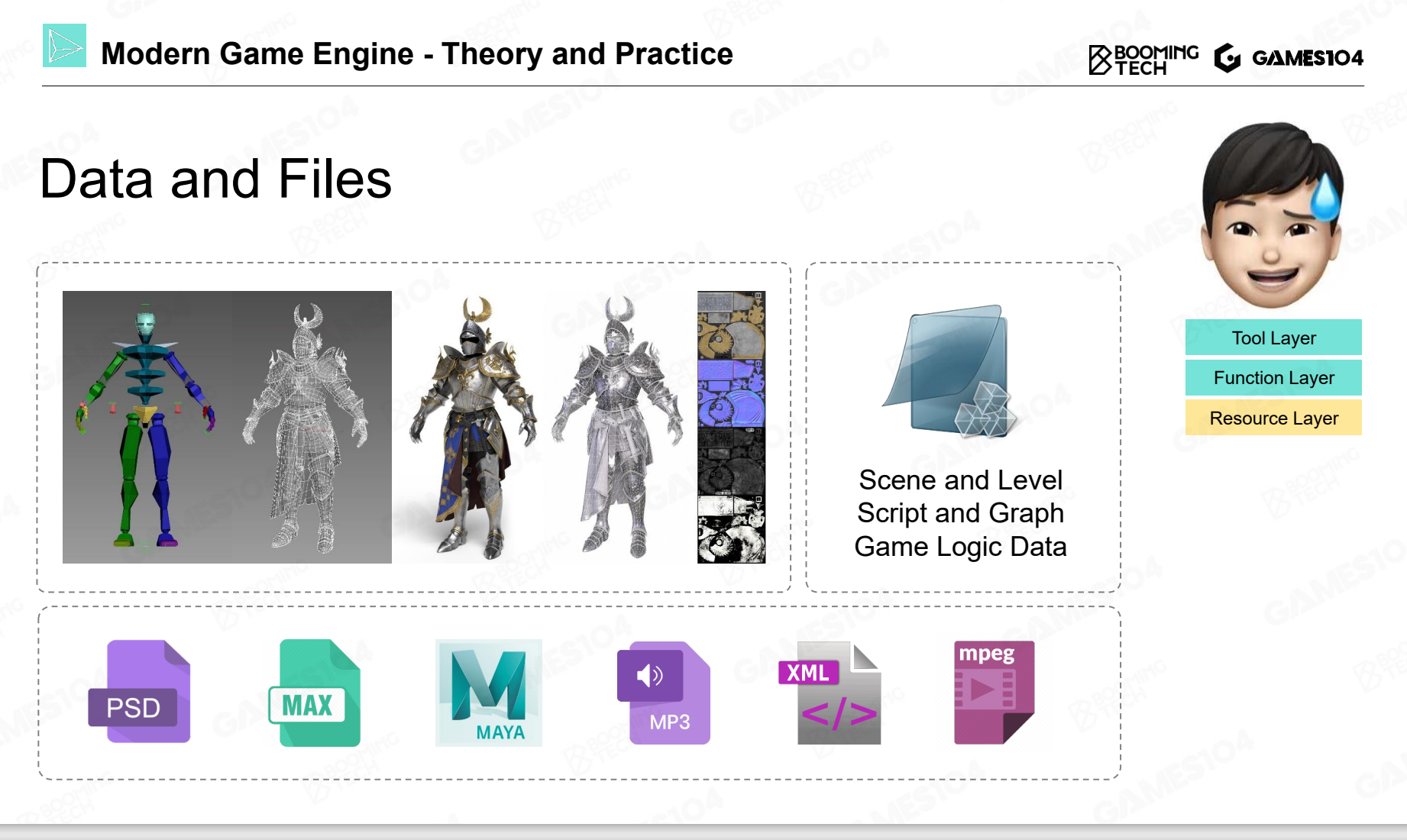
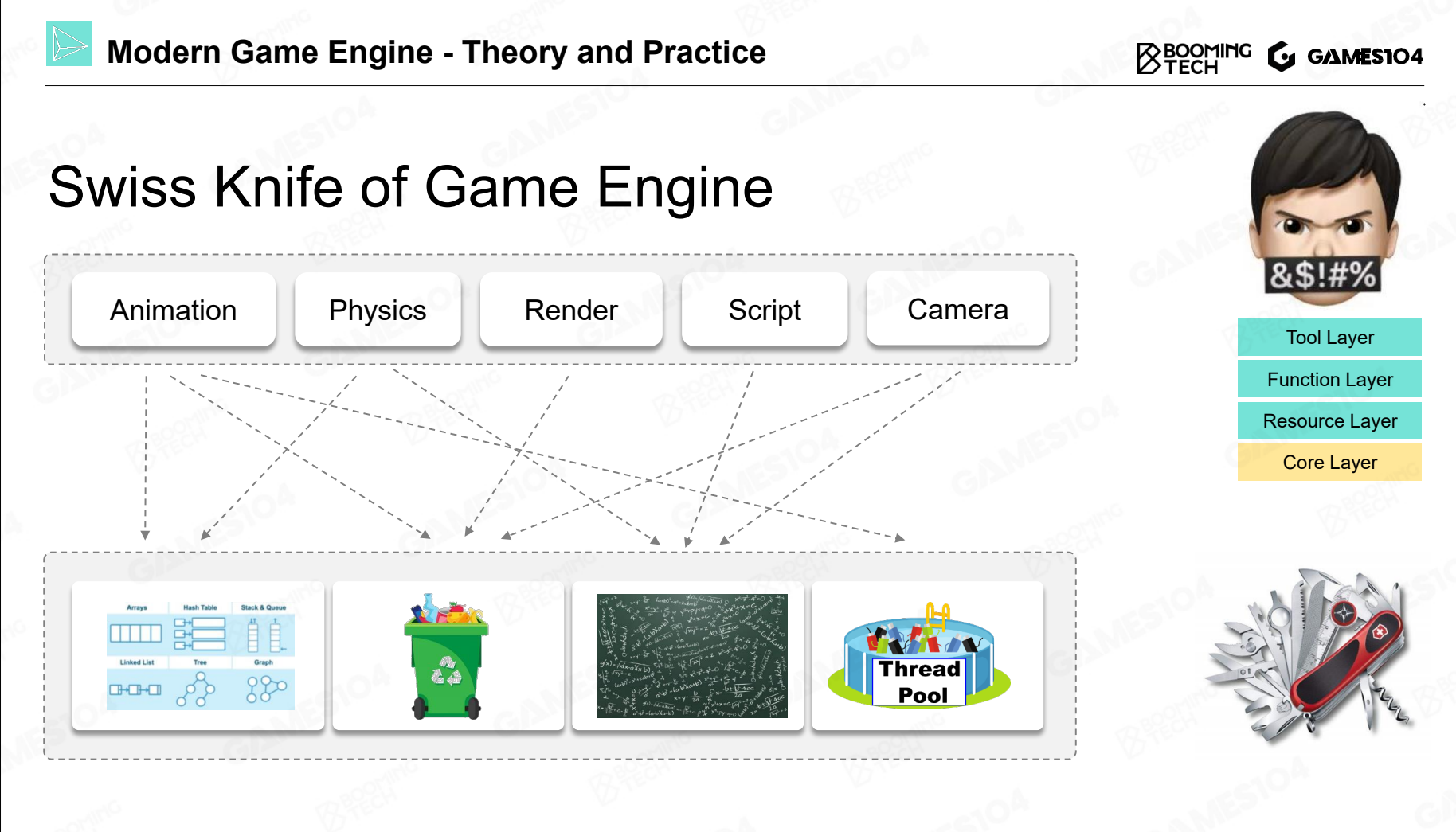
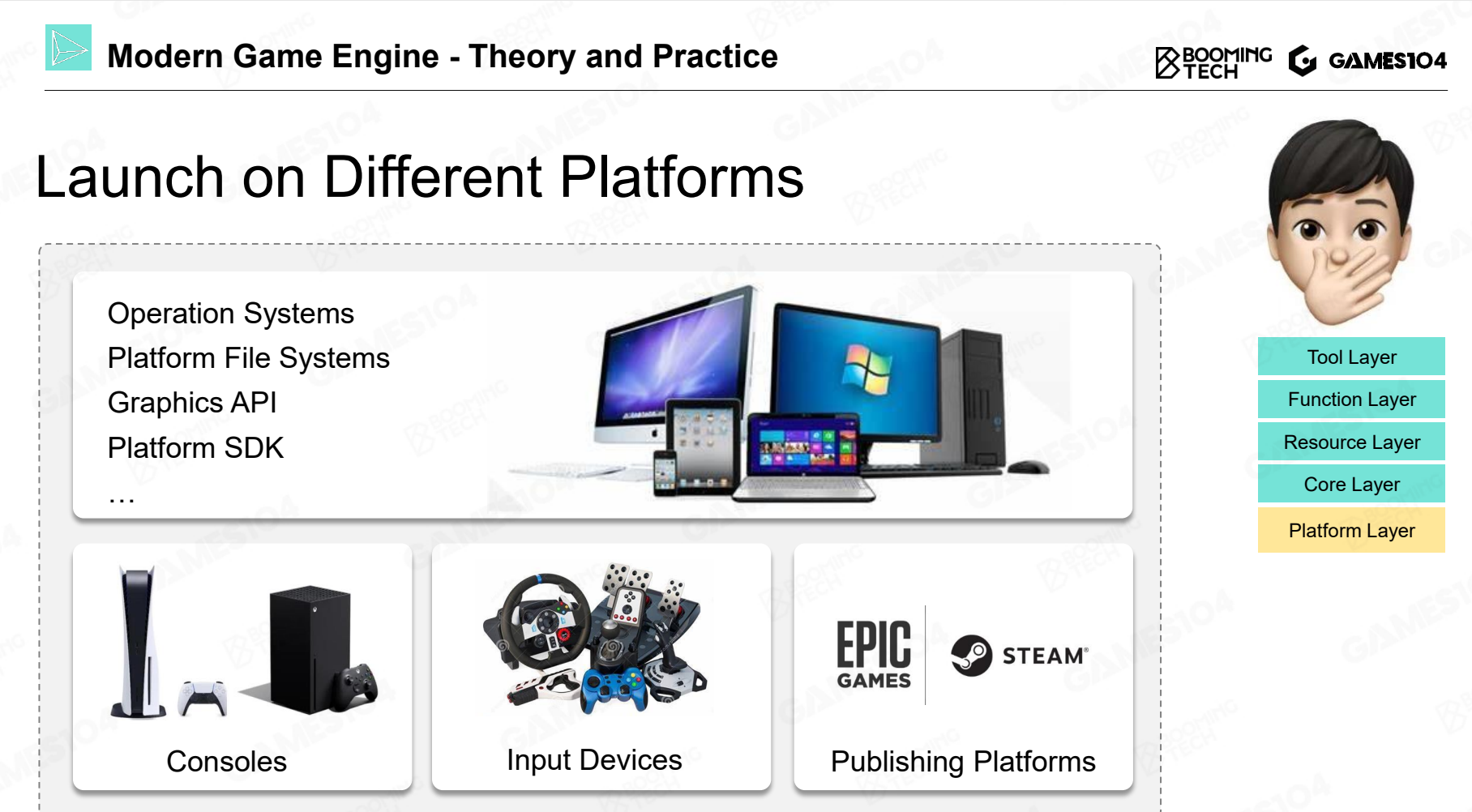
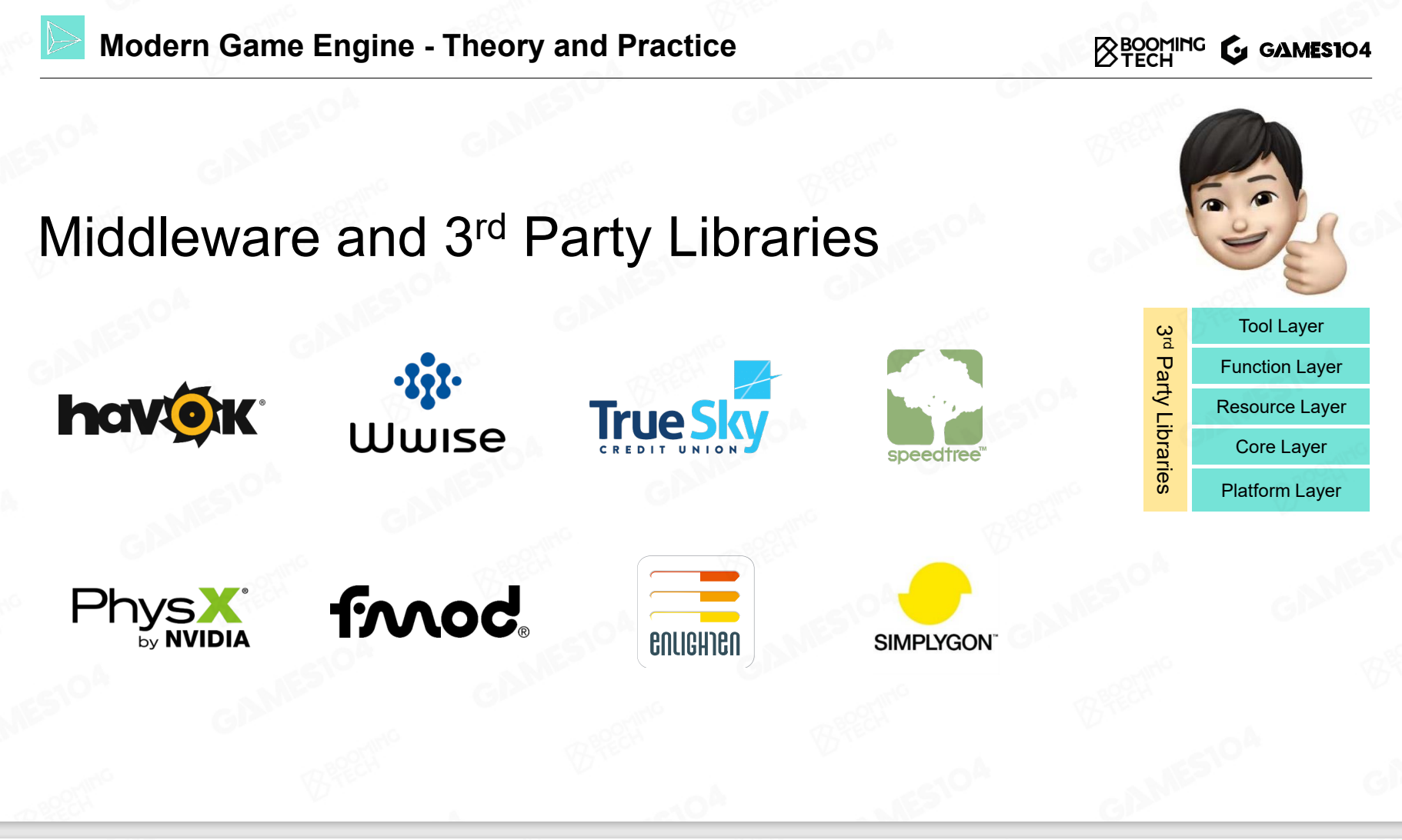
Explore Game Engine Layers
Next, we will introduce the logic and functionality of the different layers of the game engine.
Resource Layer
From Resource to Asset
The transition from resources to assets is crucial in game development. Resources refer to raw data like 3D models, textures, and animations created in tools such as 3DMax or Photoshop, stored in various formats like .max
, .psd
, or .jpg
. These formats are not directly usable or efficient within a game engine due to their complexity and storage inefficiency. To address this, resources undergo a conversion process into assets, which are optimized for the engine. For example:
- Textures in formats like
JPG
orPNG
are converted into GPU-friendly formats (e.g.,DPS
) to enhance rendering efficiency. - Large editable data from tools like Max or Maya are stripped of unnecessary information, retaining only what is essential for the game. This conversion mirrors simplifying a Word document into a lightweight TXT file. It ensures that data is streamlined for performance and compatibility, enabling smooth integration and optimal performance within the game engine. This process, termed "engine-ization," is the first critical step in turning raw resources into usable assets.
GUID - The Unique Identifier for Game Assets
In modern game engines, asset management heavily relies on establishing relationships and references among various data. For example, a robot character comprises meshes, textures, and animation assets that are inherently linked. These associations are defined in a composed asset, a relational script that specifies how these resources work together. When the engine reads this composed asset, it knows what resources to load.
To manage and track the vast number of interconnected assets in modern games—often numbering in the hundreds of thousands—GUIDs (Globally Unique Identifiers) are used. A GUID assigns each asset a unique identity, similar to a personal ID number. This ensures assets can be accurately referenced regardless of changes in their file paths or locations.
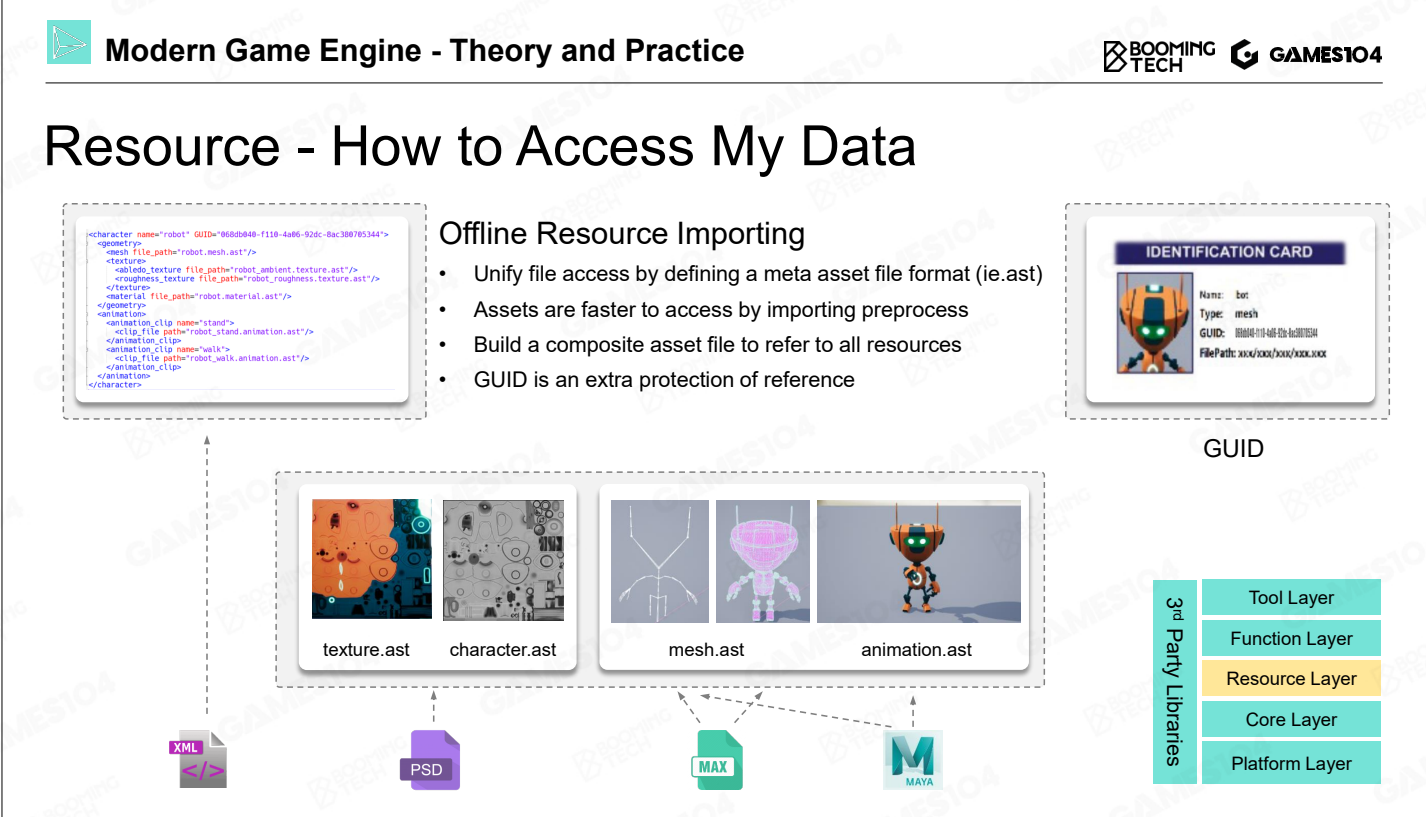
Runtime Asset Manager
When transforming scattered raw files into assets for the resource system, a runtime asset manager is essential. This system, while straightforward in concept, plays a critical role in managing the relationships and lifecycles of assets during gameplay. The Runtime Asset Manager oversees assets in real-time as the game runs. Assets are treated as interconnected files, with references linking them. The manager ensures that these links are maintained dynamically during runtime.
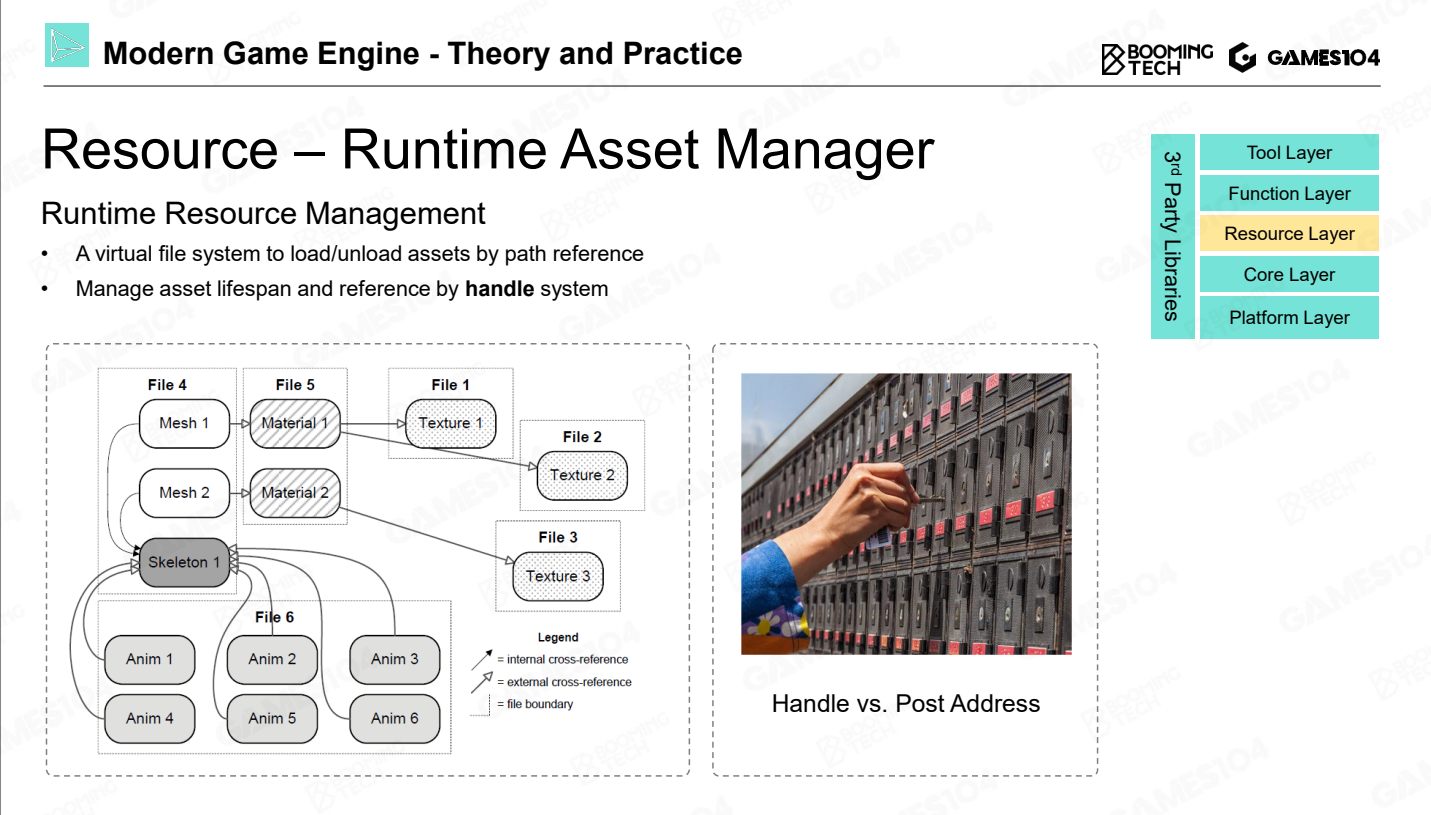
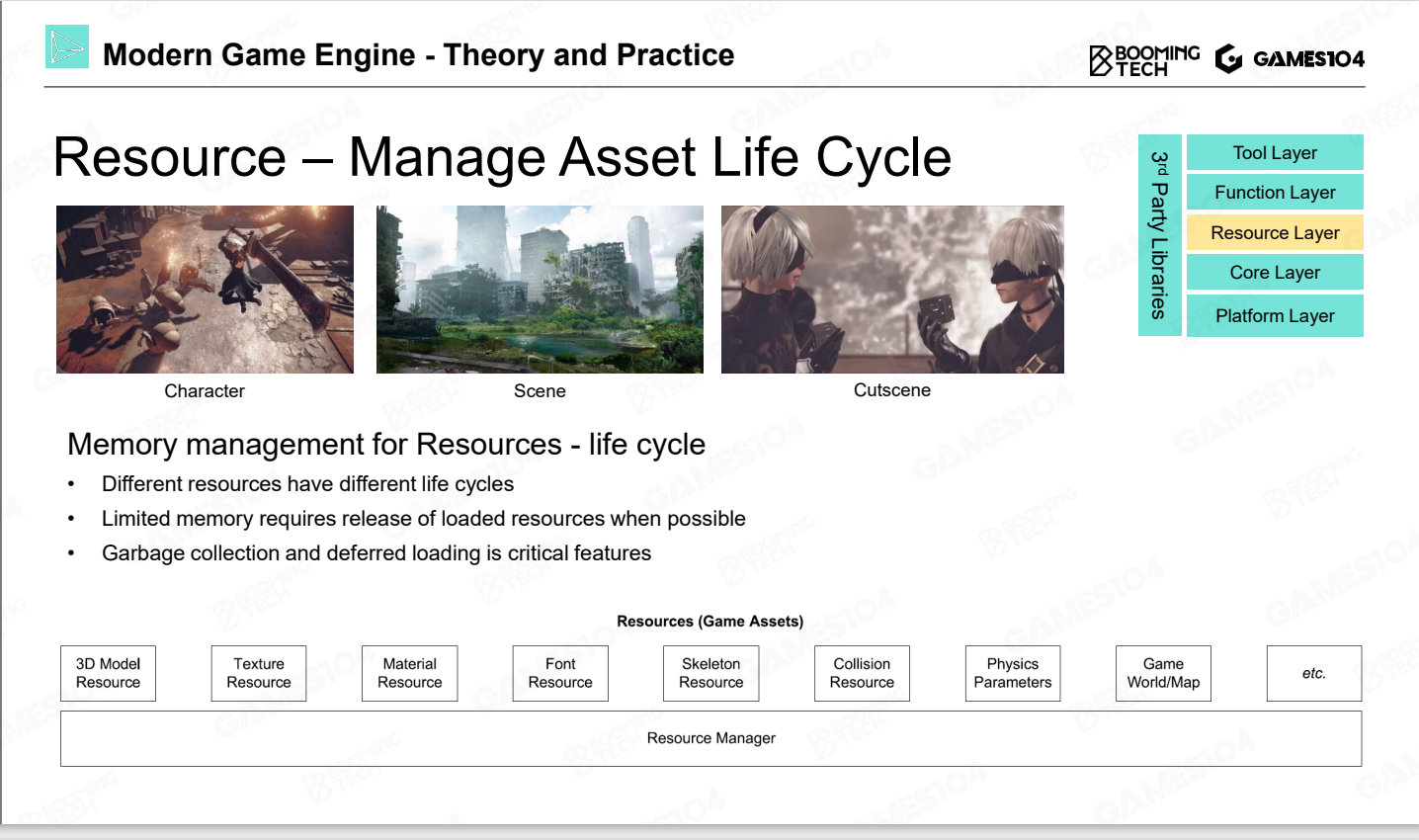
- GUID System: Ensures every asset has a unique identifier, enabling reliable referencing regardless of file location changes.
- Handle System: Acts as an abstraction layer to manage assets, similar to a mailbox system where each asset is always accessible through its "key."
Lifecycle management includes garbage collection, which cleans up unused assets. Poor GC implementation can severely impact performance, leading to frame stuttering during large-scale asset transitions, such as loading a new level.
To optimize memory usage, assets are loaded only when needed. For instance, a character’s textures in Unreal Engine might initially appear blurry before becoming detailed as higher-quality assets are loaded. This strategy balances memory and performance but requires careful design. The resource layer manages the allocation of the engine's ecosystem resource pool, handling every asset’s lifecycle in real time. This makes it a critical layer for ensuring the game engine runs smoothly.
Function Layer
The Magic of Tick: Tick Logic and Tick Render
With assets ready, the next step in game development is creating gameplay mechanics, like animating a character. To achieve this, it’s essential to understand Tick, a core concept in game engines. Tick functions as the engine's heartbeat, driving all real-time updates.
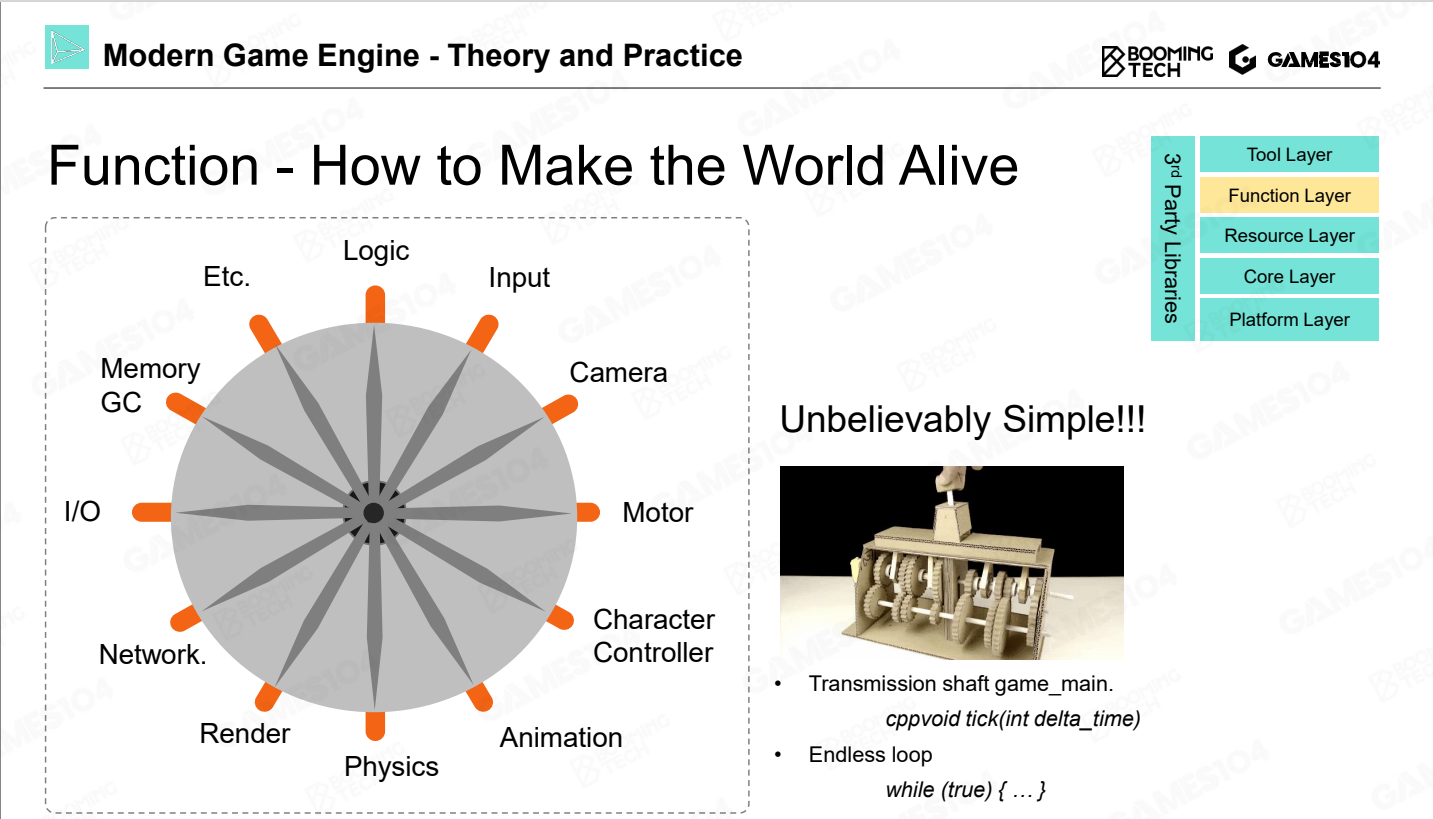
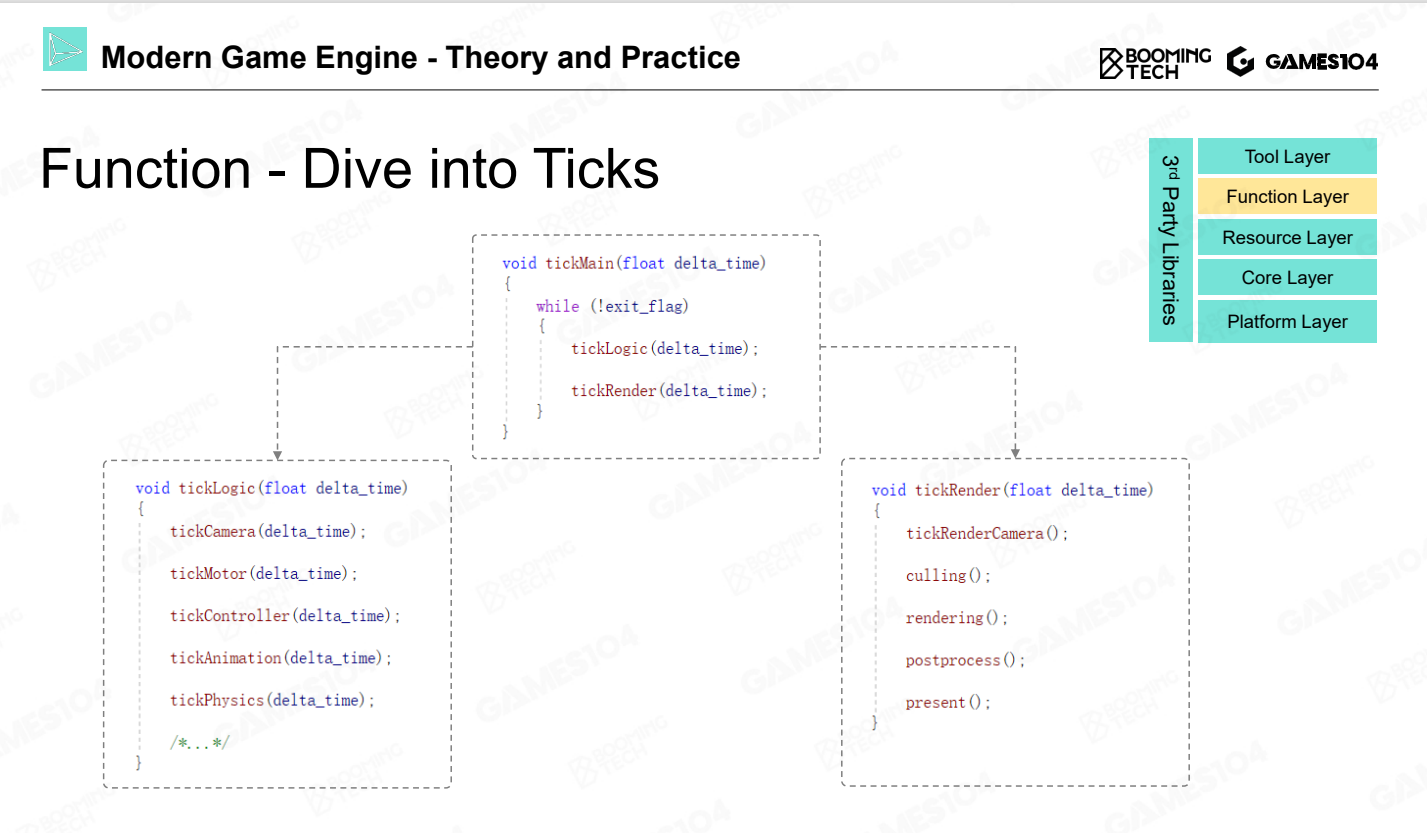
IMPORTANT
It is important to note that logic calculations always precede rendering
Tick Logic simulates the game's world by calculating physics, updating object states, and managing interactions. It operates independently of rendering, ensuring that the state of the world is consistent, whether or not it is being displayed. For example, determining whether one character hits another or updating a player's position based on input is part of this process.
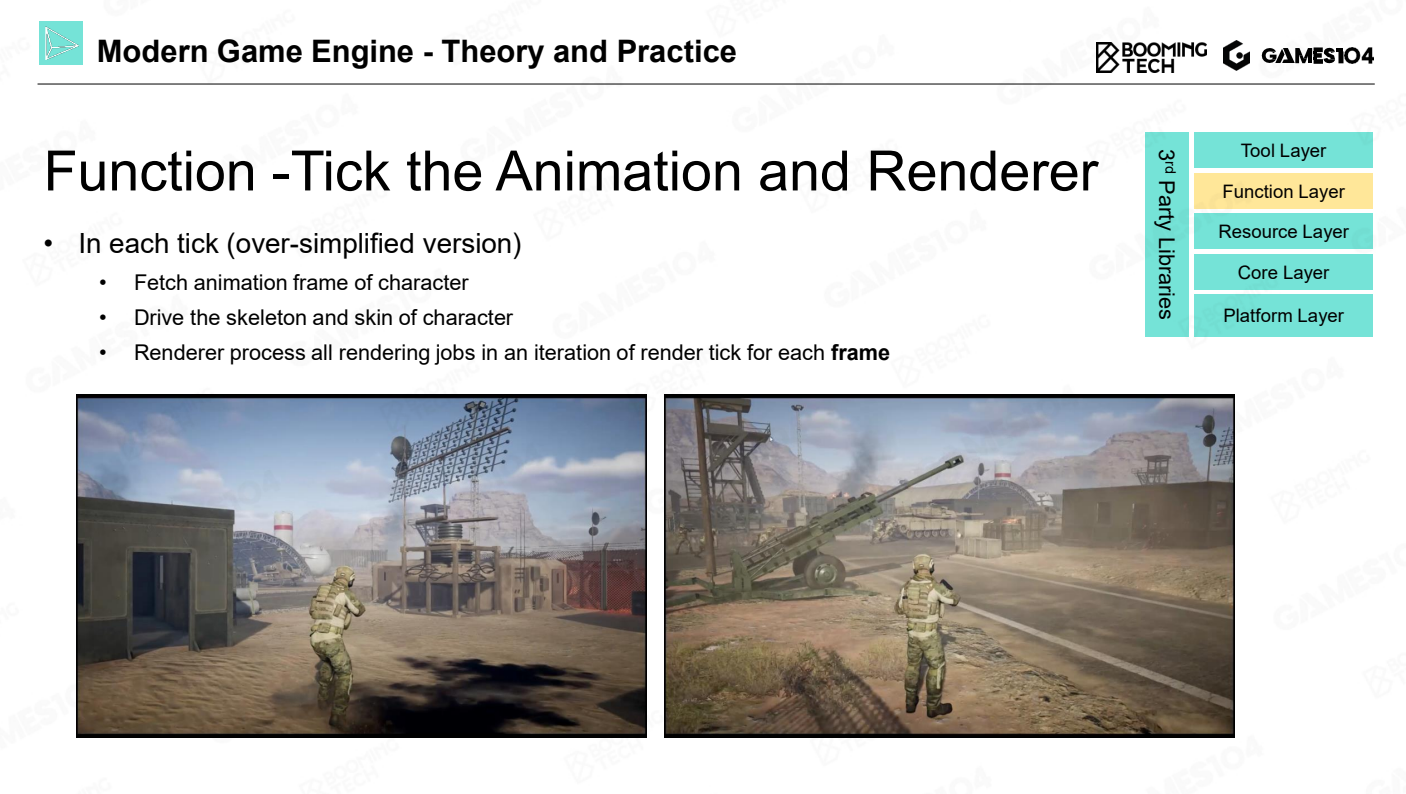
we only covered one functional layer, focusing on the simplest concept: the progression from Logic to Render. However, the functional layer of a game engine is vast and multifaceted. Referencing the classic text Game Engine Architecture, the functional layer is one of the most expansive topics in game engine design, encompassing nearly every aspect of game functionality.
Some modules, like rendering pipelines, are clearly part of the engine. These foundational components are integral to the engine’s architecture and serve all games built on it. However, other modules blur the line between the engine and specific game implementations. For instance, camera control is a particularly contentious example. While a game engine may provide basic camera rendering capabilities, the nuanced behavior of the camera—such as the handheld shakiness in a third-person shooter, motion blur, or dynamic zooming—often ties directly to the gameplay experience.
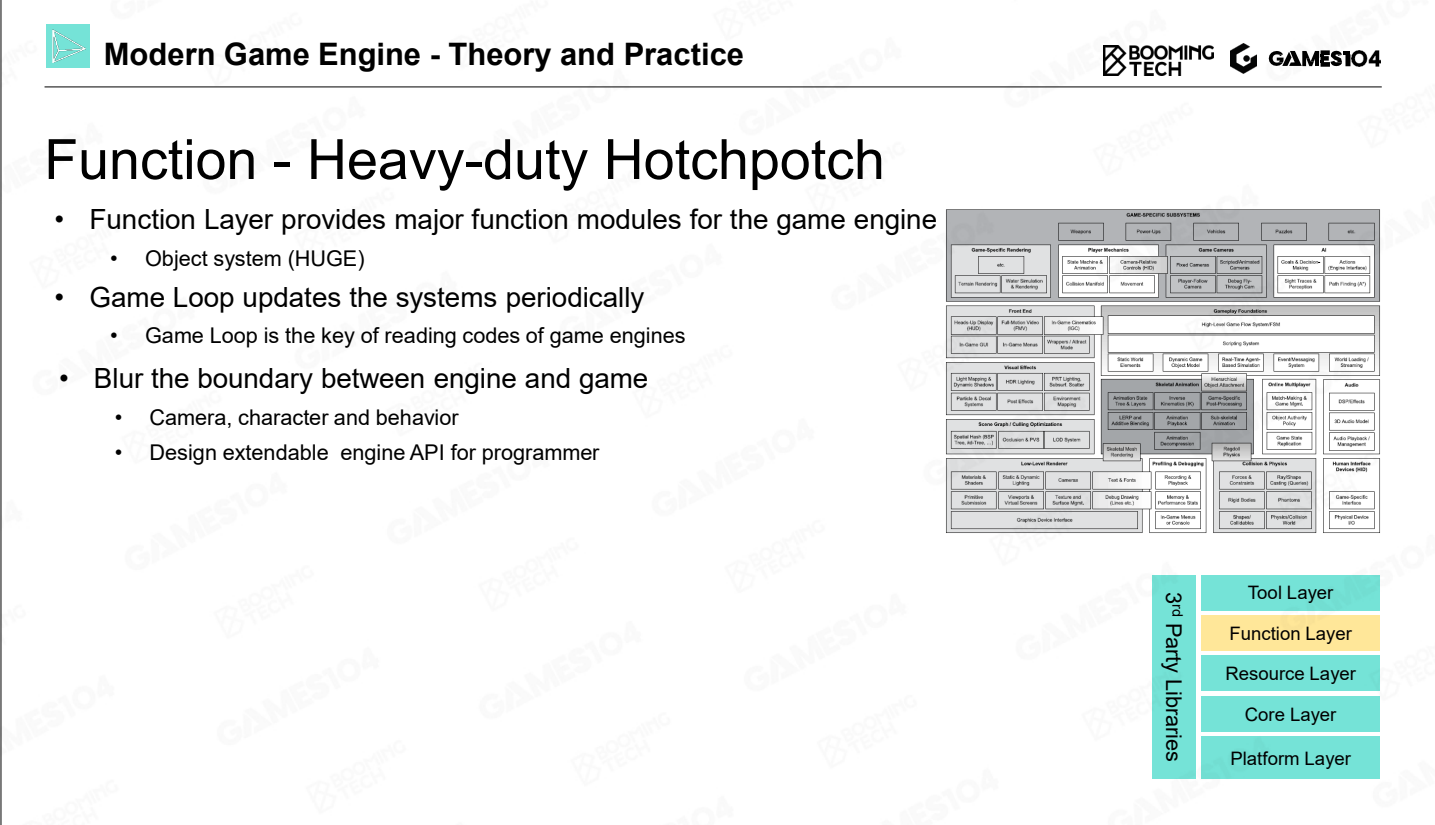
Multicore Era in Game Engine Architecture
Modern computer architecture has transitioned from single-core to multicore processing, fundamentally shaping game engine design. Early game engines were single-threaded, with all tasks running sequentially. As multicore processors became standard, engines adopted multithreading, initially separating tasks like logic updates and rendering into different threads, alongside additional threads for operations such as asset loading.
Commercial engines like Unity and Unreal have advanced this further, isolating easily parallelizable computations—such as physics simulations and animations—into separate threads. This evolution leads to architectures capable of distributing tasks across multiple cores more effectively. The future lies in breaking tasks into atomic units, often referred to as jobs, which can be dynamically assigned to any available core. This approach maximizes CPU utilization, regardless of the number of cores, ensuring each core is consistently active.
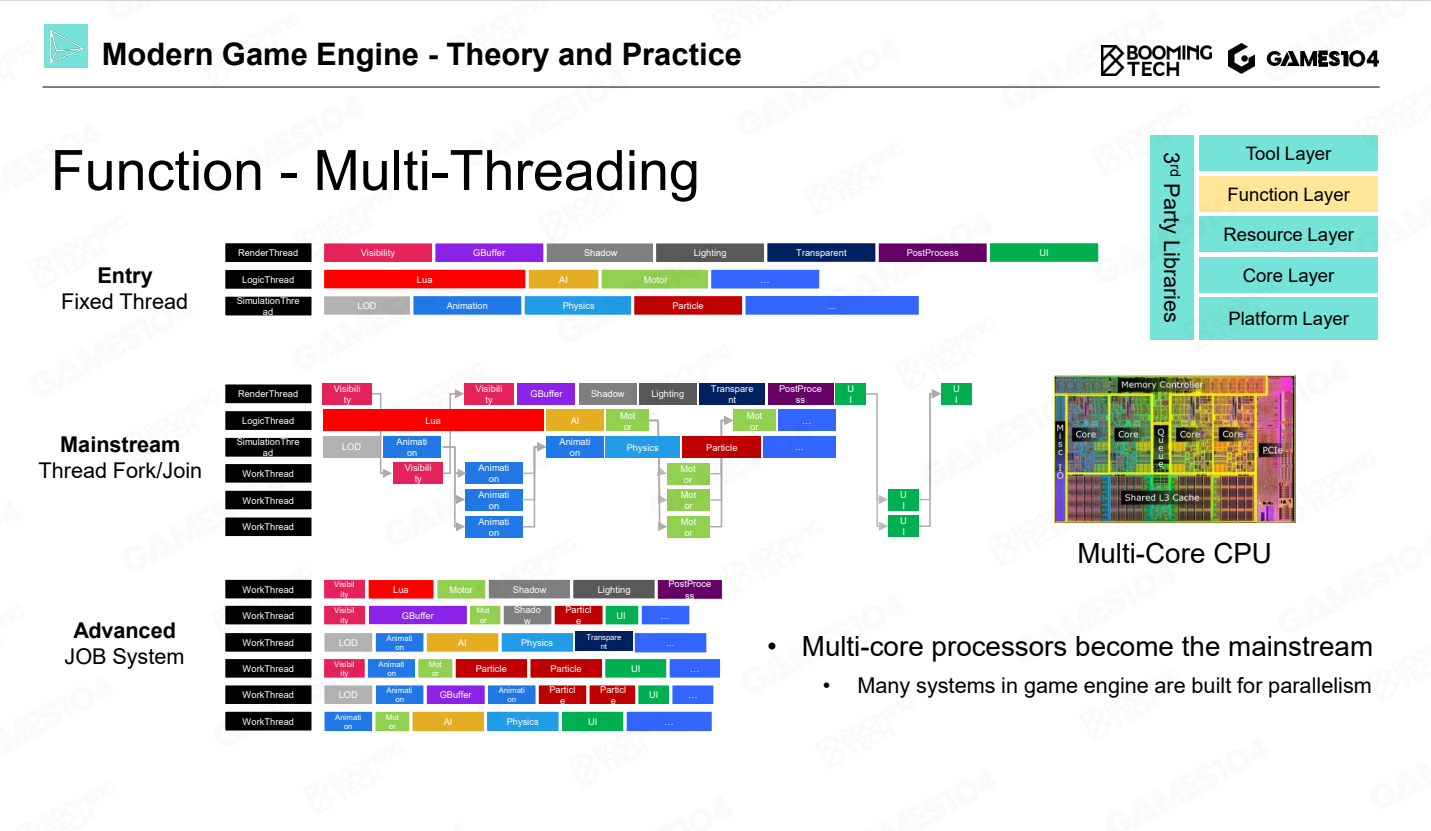
Core Layer
In the core layer, the math library often draws the most attention. It serves as the foundation for essential computations in the game engine
Math Library
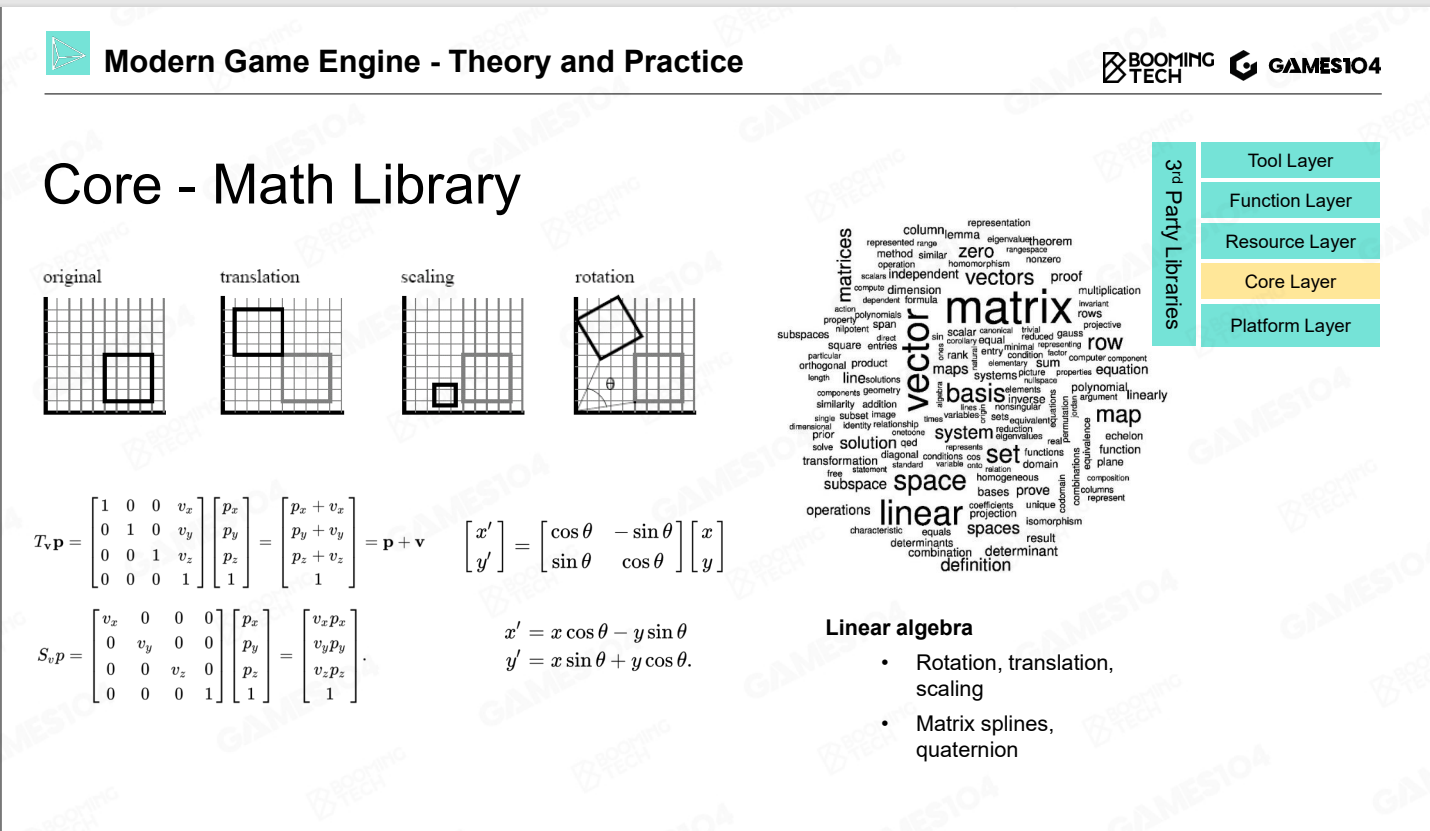
In game engines, much of the mathematical computation revolves around matrix and vector addition, subtraction, multiplication, and division. A key optimization for these operations is SIMD (Single Instruction, Multiple Data). SIMD allows a single instruction to process multiple data points simultaneously—such as multiplying or adding four numbers at once.
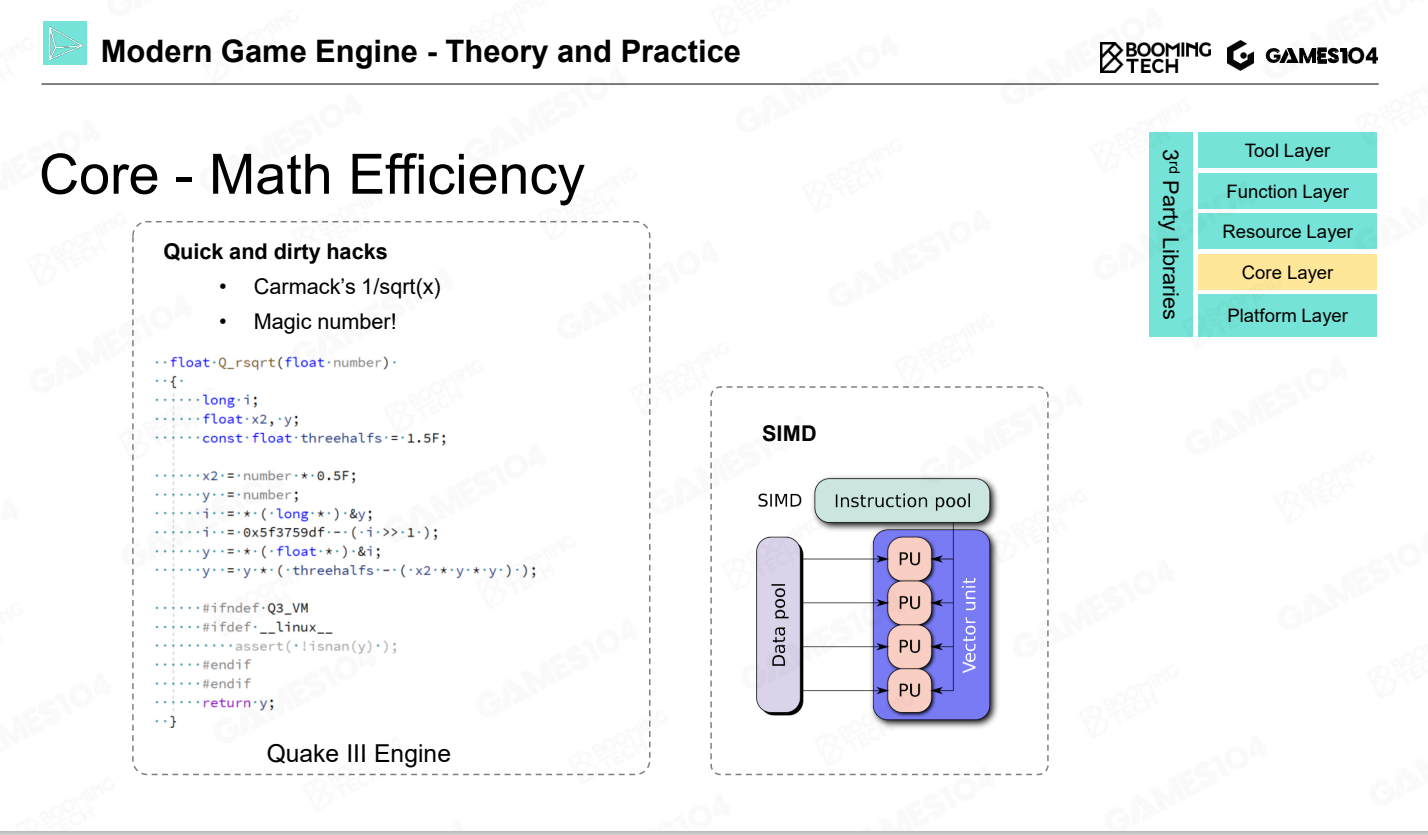
Data Structure and Memory management
Beyond the math library, the core layer must also include implementations of various commonly used data structures. While the C++ Standard Library (STL) provides a broad range of data structures, some of its implementations are not optimized for the specific performance requirements of game engines.
Here’s an interesting detail about Vector, a common data structure. In the standard C++ implementation, when a vector exceeds its initial capacity (e.g., from 100 to 101 elements), it typically resizes by doubling its allocated space. While this approach minimizes resizing frequency, it has drawbacks.
During resizing, the vector allocates new memory, copies existing elements to the new location, and releases the old memory. This process can lead to unpredictable memory consumption and fragmented allocations, especially in a resource-intensive game. Fragmented memory slows down access and degrades overall performance.
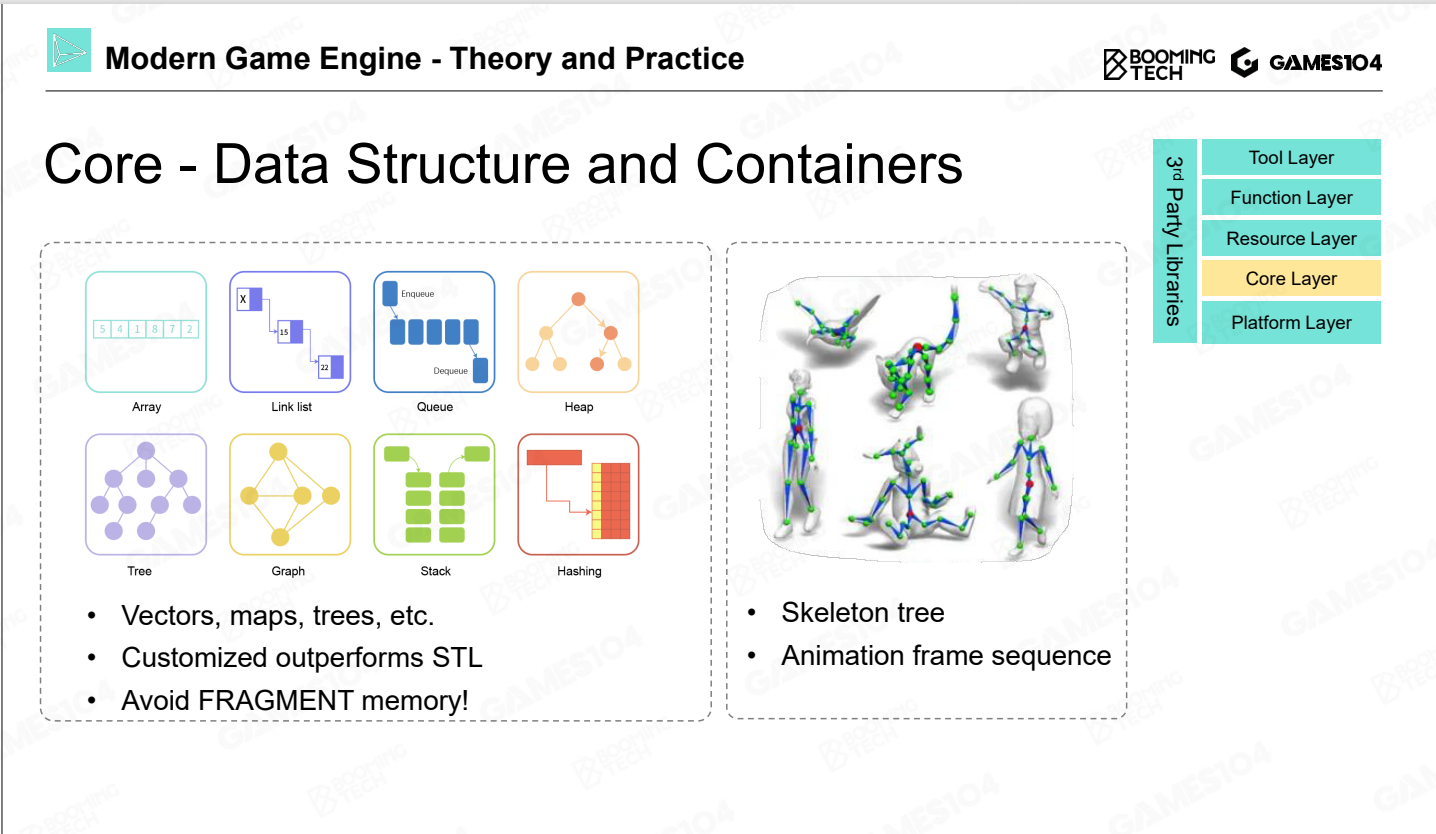
Memory management is one of the most critical functions of a game engine’s core layer. As discussed earlier, building a game engine closely resembles creating an operating system, where managing memory is a fundamental task. In game engines, this involves pre-allocating a large block of memory and managing it internally to maximize efficiency. In the core layer, memory management typically involves using a memory pool to manage all memory resources collectively, including the allocation of various data structures such as vectors and lists.
These concepts might seem abstract, but they're actually quite simple to understand.
The Turing machine, invented by the legendary Turing, has a straightforward structure: it's essentially a perforated tape carrying code. By pulling the tape back and forth and performing read and write operations, it can execute all logical tasks. To improve the efficiency of such a machine, data management needs to follow some basic principles.
The core ideas are: group related data together to facilitate faster sequential access; access data in a logical order rather than randomly jumping around; and handle data modifications in batches rather than individually.
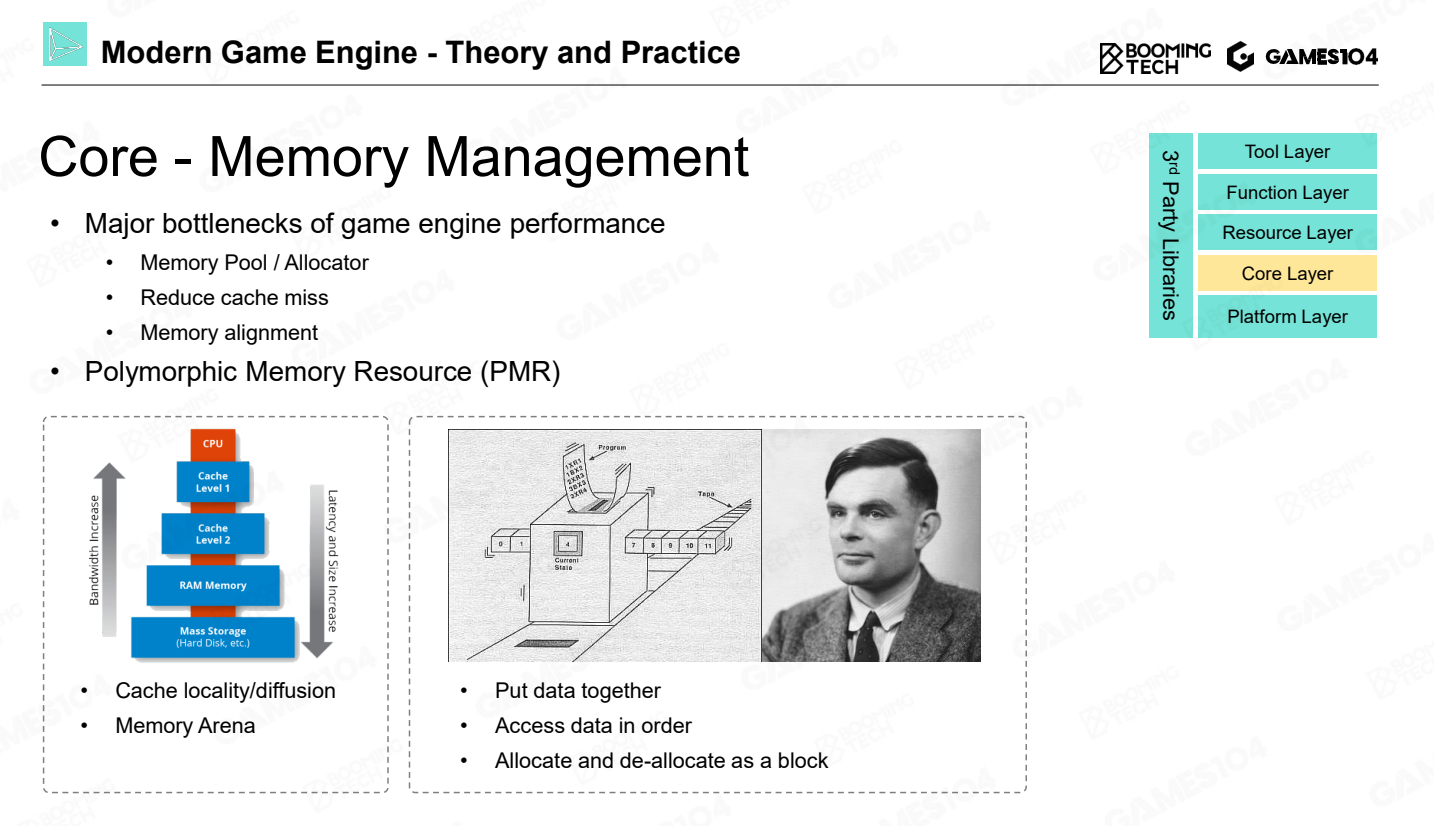
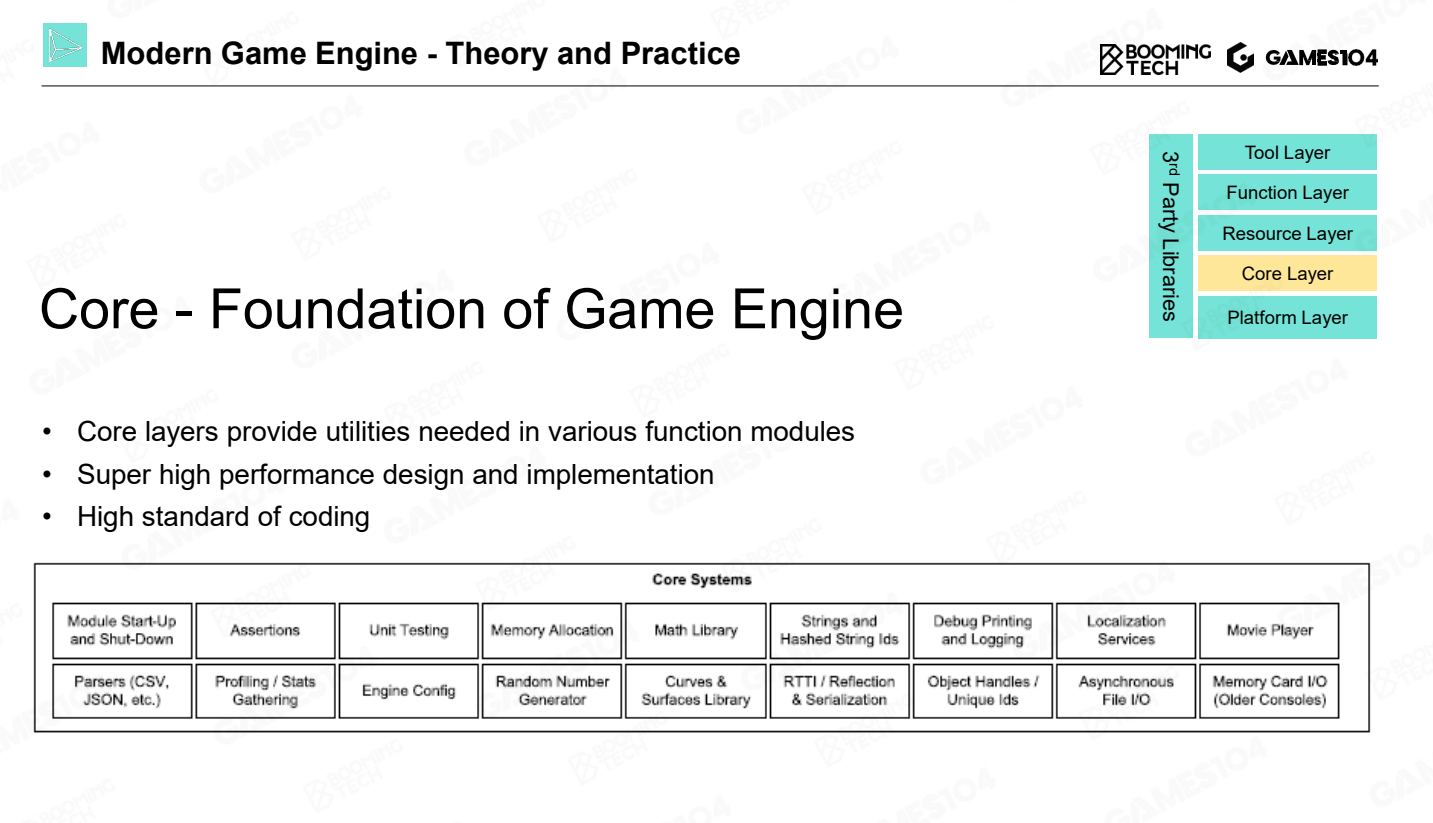
Platform Layer
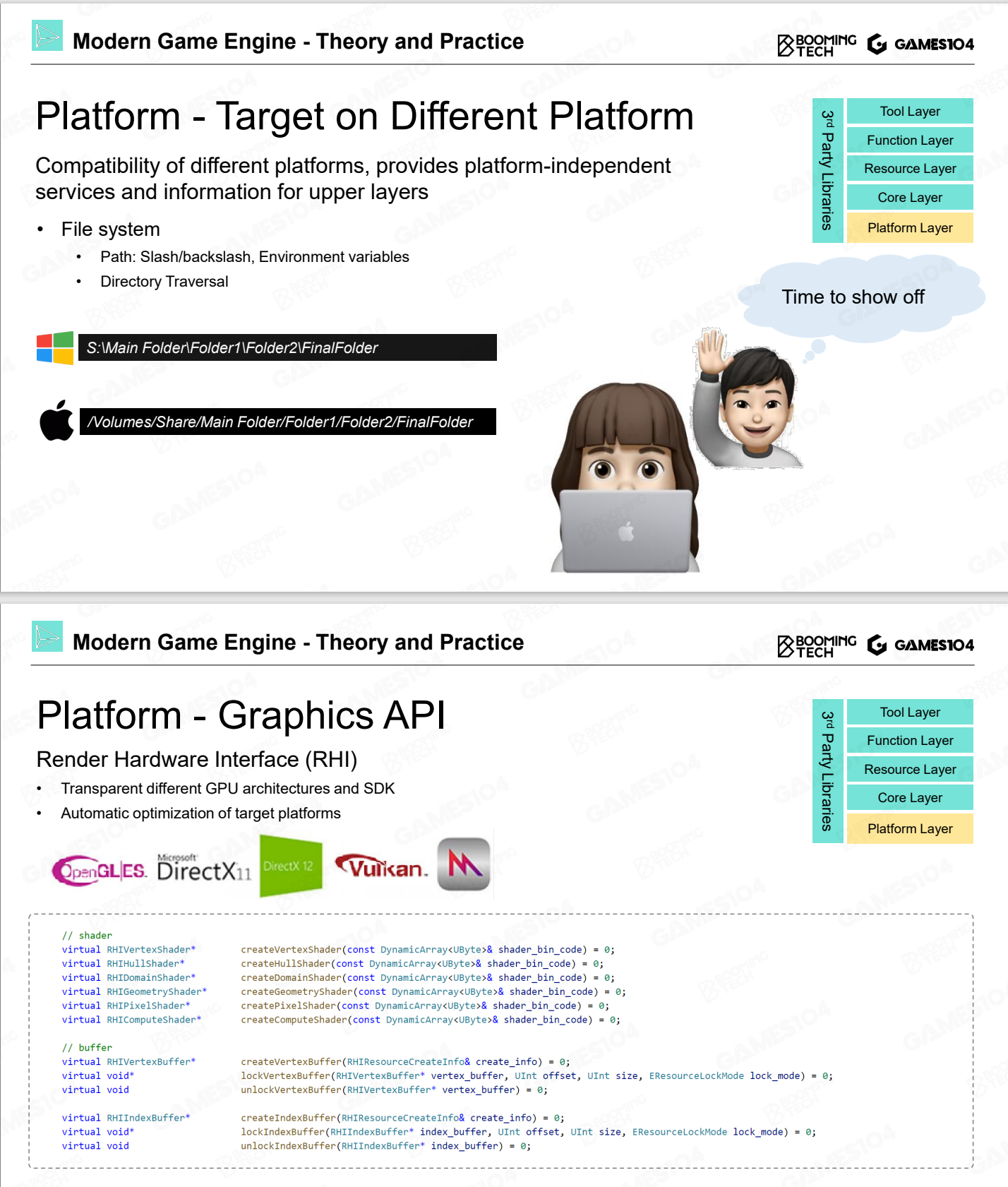
Tool Layer
A game engine can be divided into two main components: the real-time runtime layer and the tools layer. The tools layer is discussed last because it isn't part of the runtime.
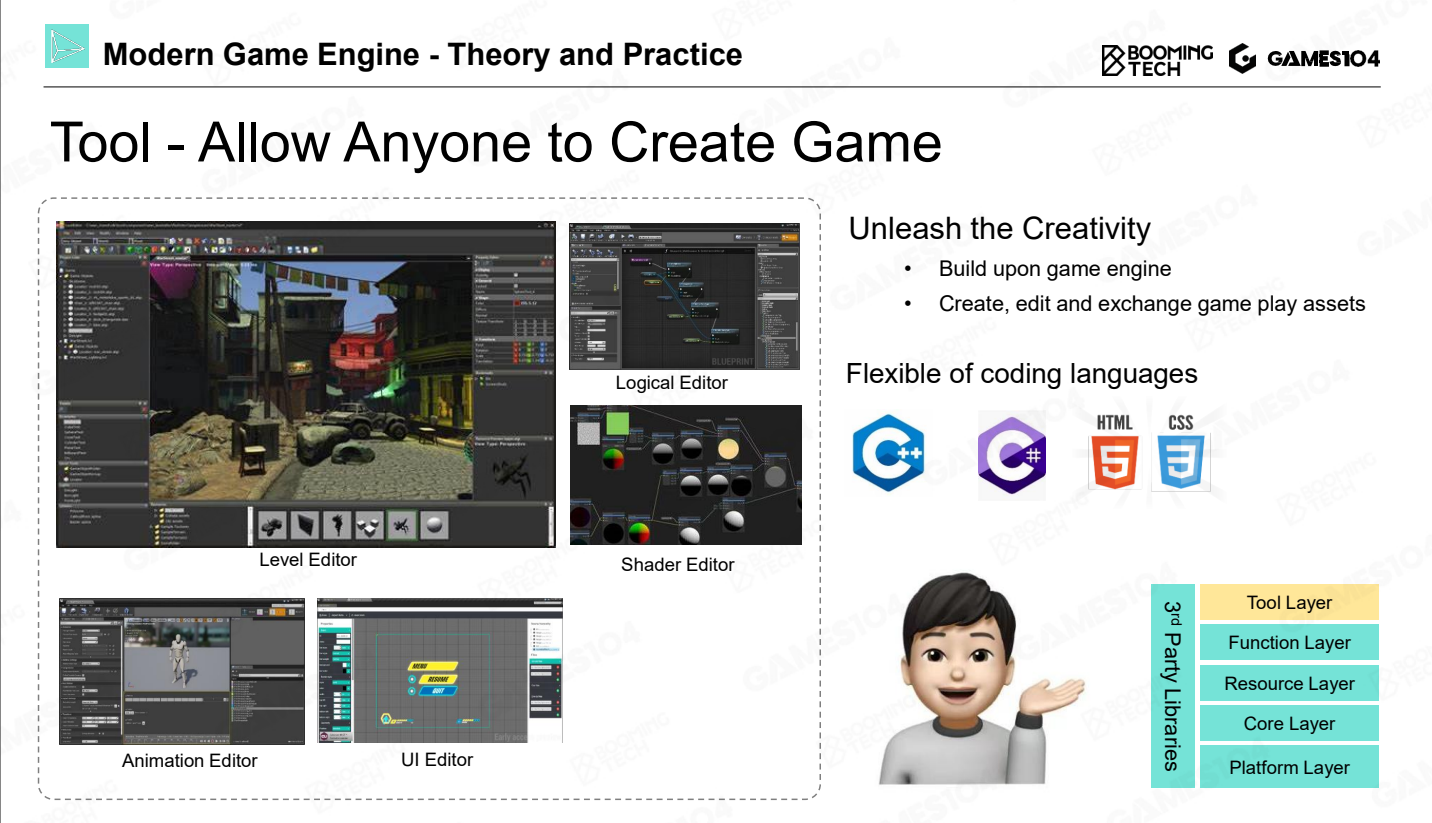
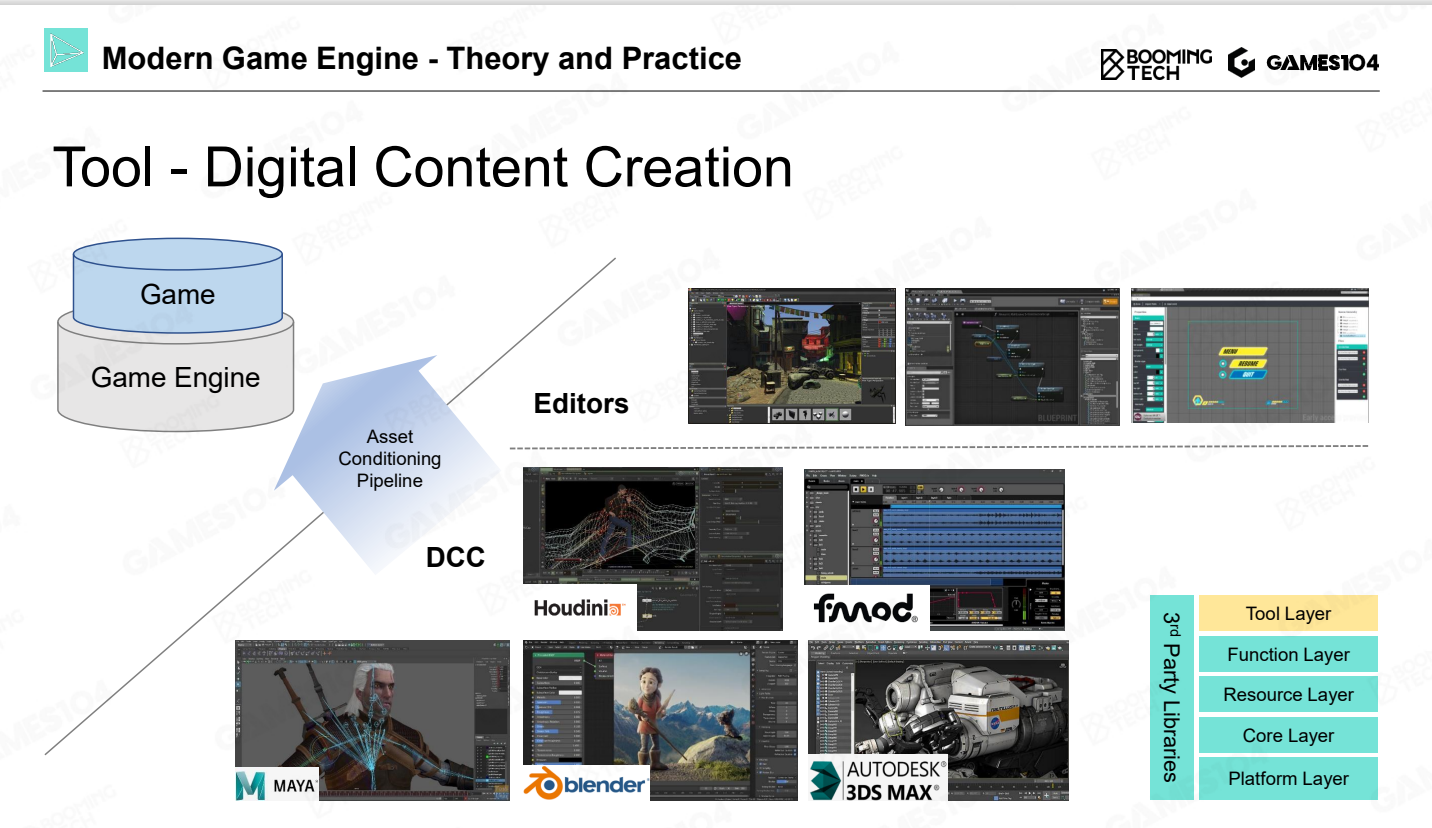
Why Layered Architecture?
The purpose of layering in a game engine is to decouple different types of code, enabling better management of the system's complexity.
NOTE
In system architecture, a foundational principle is that the deeper the layer, the less it should be modified.
For example, the platform layer is highly stable, with its code rarely changing over the years. In contrast, the functionality layer can be adjusted more frequently, while the tools layer evolves almost daily to accommodate new features or tools. This hierarchy ensures flexibility at the top and stability at the bottom, which is a key advantage of layered structures. When addressing new requirements, it’s essential to identify the appropriate layer for the task rather than hastily implementing algorithms.
Aspiring game engine developers often focus on creating specific features with exceptional quality, but systematic training emphasizes the importance of understanding which layer a feature belongs to.
IMPORTANT
A critical practice in layered architecture is restricting inter-layer calls:
upper layers can call functionalities in lower layers, but lower layers should never call upwards.
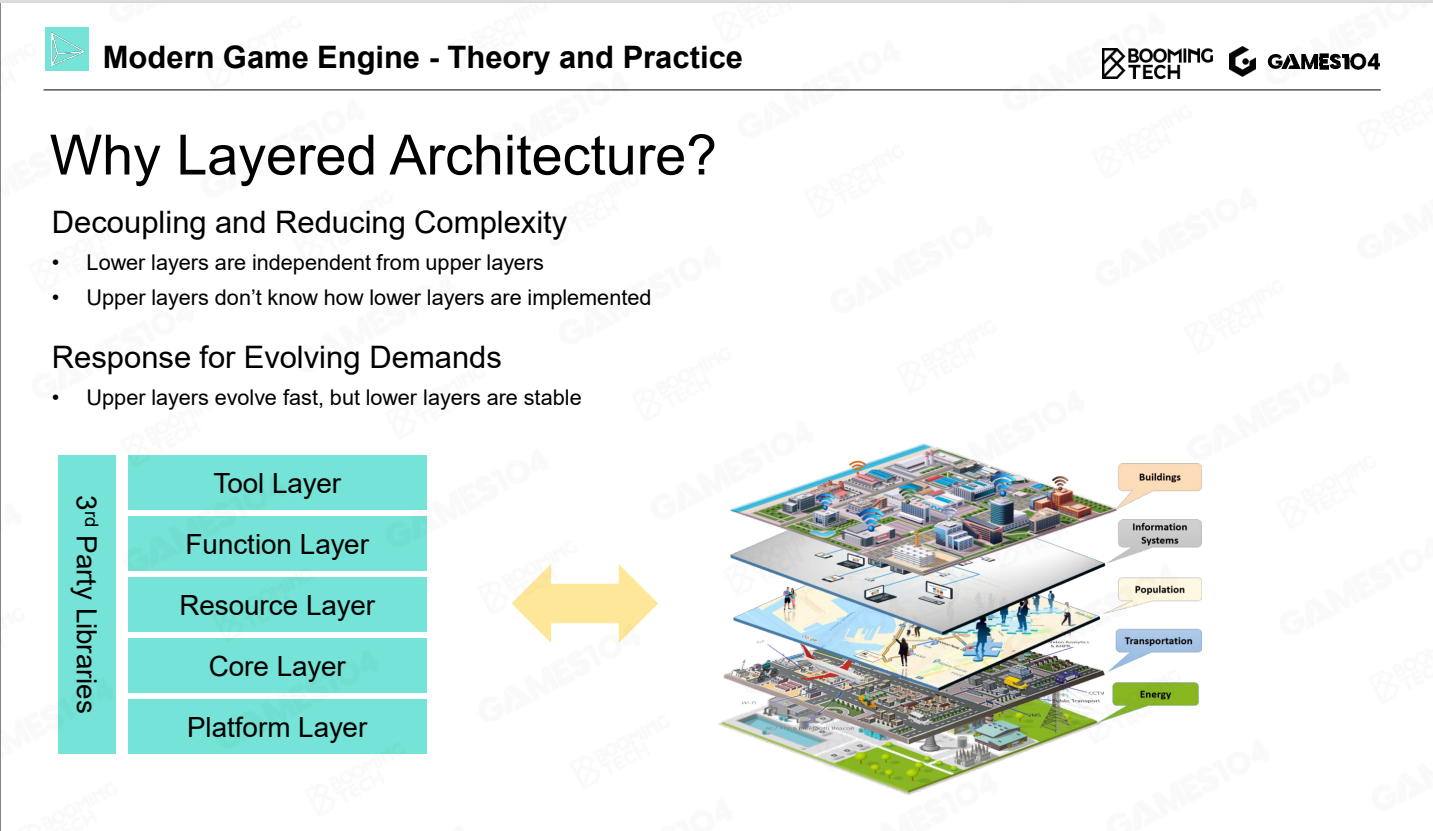